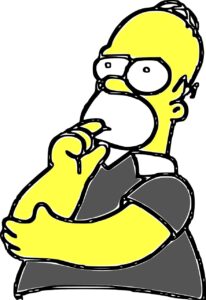
Typescript is a compiled and strict type programming language that is built upon Javascript. Or we could say it’s a superset of Javascript. Do you understand this? It’s more of a technical definition, isn’t it? Yes. Let’s crack it in more simple and in basic terms what exactly it is.
Typescript was developed by the developers team at Microsoft led by Anders Hejlsberg who also developed C# and contributed to some programming language development. But why?! Why?! Why?! Why?! Weren’t we happy with Javascript?!
Well, that’s not the catch. The main aim was to make Javascript more collaborative and more safer in terms of type. Yeah yeah i know. It’s getting more technical. Let me crack it for you in simple words.
We know that Javascript value holders or we can say variables can hold any value without specifying its type. It’s what we know as dynamic typing. Dynamic typing results in runtime errors and it’s very hard to catch these errors during the development of large and complex applications.
Typescript added the solution to these issues by adding static and strict type checking reinforcements along with other sets of new features such as enums, interfaces, classes and enhancements to write more modularized, robust, powerful and error-less code.
Is javascript the same as typescript?
The primary answer is No. It’s not a huge and confusing term for normal developers. But if you are a beginner and learning typescript without the basic understanding of Javascript you might struggle a bit to justify the actual difference. It’s not that hard. But the primary answer is: No.
Javascript is a highly interpreted and dynamically typed language. As we discussed above what exactly dynamically typed is, Typescript is a highly static type programming language. It means that it focuses more on type as its name suggests which makes it more stronger than Javascript. Well it’s theory and it’s kind of boring, I know. Without an example you won’t understand it, so here it is.
Javascript:
function addVariablesValue(a, b) { return a + b; } console.log(addVariablesValue(2, "4"));
Typescript:
function addVariablesValue(a: number, b: number): number { return a * b; } console.log(addVariablesValue(2, "4")); // TypeScript error: Argument of type 'string' is not assignable to parameter of type 'number'
As you can see above, Javascript will dynamically assign the type to addVariablesValue arguments and then perform the operation. Since one argument is number and another is string it will dynamically assign a type and perform the operation and we will lead to desirable results. While on the other side in typescript, it already specifies that it will not allow other than specified data types for arguments and generates an error while providing an un desirable input.
The preservation and enhancement of Javascript traditions
“Typescript is a superset of Javascript.”
Okay, I might repeat this statement again and again. But what exactly does it mean? If you look at it in technical terms, it’s built on the of javascript. Means that typescript has all the features of javascript. Also it does come with a new set of features such as static typing, generics and interfaces, Type interfaces, and many more features.
In real terms, actually typescript code cannot be executed directly. First it needs to convert into javascript so that browsers and javascript engines can run it and then it will be executed further for targeted operations and results. Static analysis and static typing run at compile time.
Enum:
Enum in is one of the enhanced features added in typescript unlike in Javascript. Enums make the code more readable and add more type safety to the code. Enum does define a limited set of types which enforces the type safety. Enums are also smart as if you don’t define the values of the data inside the enum then it will automatically assign values.
enum Roles { SuperAdmin, Admin, User, }
Here superAdmin will refere to 0, Admin will refere to 1 and User will be refere to 2 as default assinged value.
Literal types
Littral types in Typscript are one of the core and powerful features that help to define and use more established, effective, restricted and precise type.
let answer: "yes" | "no"; // Value of answer can only be either yes or no answer = "yes"; // Valid answer = "no"; // Valid answer = "none"; // Error: Type '"none"' is not assignable to type '"yes"' | '"no"'
Here, you can see we assigned specific types using literals to the variable “answer”, so “answer” can now only have two values, either “yes” or “no”. It will accept only two values. if we assign other than that it will throw an error and will not allow us to go through the execution.
There are so many other enhanced features that have been introduced such as interfaces and typed interfaces and many more. So typescript does maintain the traditional javascript programming structure and on top of that typescript has been built so it has all the access to javascript and along with the new features of typescript. Hence why it’s a superset of javascript.
Bug detection and collaboration
The type checking process occurs during compile time, so bugs can be identified and fixed before execution, enabling bugless code. It is just good for bug detection. Compile time error handling also results in clean and neat code.
Clear and properly defined types help developers to read, write, modify and execute it perfectly. This is common and pre-established structure of the code that helps to understand the code properly and improve the code understanding which leads to team collaboration and many other benefits.
In other words, typescript enhances javascript by adding new features and it runs on top of it, so it has all the core features of javascript.
How to install and use Typescript?
Typescript code cannot be executed directly. The code needs to be converted into traditional Javascript code and then the javascript engine and browser can understand it. So for that we need a typescript compiler. So to install and write your first typescript code, follow the below down process.
You can “install typescript” using the npm command.
"npm install -g typescript"
After installation, create the file with the .tsx extension, write down your own typescript code and save it. Now open your command line and run tsc youfilename.tsx and hit enter. it will generate you translated .js file.
tsc youfilename.tsx
To check out in the browser, create index.html file, add this file in the header section of your html code,
<head> ------- ------- <script src='youfilename.js'></script> ------- ------- </head>
Compile the code using “tsc youfilename.tsx” and run the html file in a browser and check the results.
Summary
Since Typescript is built entirely on top of Javascript, as well as having many new and enhanced features, it’s called javascript’s superset. Unlike JavaScript, which is dynamically typed, TypeScript enforces type checking at compile time, reducing runtime errors, especially in large projects. Enums and literal types have been added to the for more enhanced and stronger type safety. It has better code readability and collaboration compare to Javascript.